Serial point calculation Tutorial
First open a terminal and execute Julia, then type using MAGEMin_C
to load the package.
Initialize database
# Initialize database - new way
data = Initialize_MAGEMin("ig", verbose=true); # database: ig, igneous (Holland et al., 2018); mp, metapelite (White et al 2014b); mb, metabasite (Green et al.,2016); um, ultramafic (Evans & Frost 2021)
This initiatizes the global variables and the Database.
Set P-T-(pressure temperature)
P = 8.0
T = 800.0
use_predefined_bulk_rock
retrieves the saved bulk-rock composition 0 from database ig, which corresponds to KLB-1 peridotite.
Set bulk-rock composition
Use a pre-defined bulk-rock “test” composition
or a custom bulk-rock composition:
Xoxides = ["SiO2"; "Al2O3"; "CaO"; "MgO"; "FeO"; "Fe2O3"; "K2O"; "Na2O"; "TiO2"; "Cr2O3"; "H2O"];
X = [48.43; 15.19; 11.57; 10.13; 6.65; 1.64; 0.59; 1.87; 0.68; 0.0; 3.0];
sys_in = "wt"
Note that the system unit [mol,wt]
has to be provided here.
where Xoxides
is a Vector(String)
containing the oxide names and X
is a Vector(Float)
of the [mol,wt]
fraction of the bulk-rock composition.
The function converts
SiO2
, …,FeO
andFe2O3
in system unit[mol,wt]
to:
SiO2
, …,FeOt
andO
in system unit[mol]
.
Note that if the provided bulk-rock composition includes more oxides than supported, they will be ignored and the composition will be renormalized accordingly. Moreover, if both Fe2O3
and O
are provided, O
will be recalculated as function of Fe2O3
. Thus, if you want to prescribe a different O
content, do not define Fe2O3
!
Call optimization routine for given P-T-X
out = point_wise_minimization(P,T, data);
if a predefined test is used (see List of pre-defined bulk-rock composition) or:
out = single_point_minimization(P, T, data, X=X, Xoxides=Xoxides, sys_in=sys_in)
if a custom bulk-rock composition is provided.
Display minimized point
@show out
The command @show
allows you to display the minimized point:
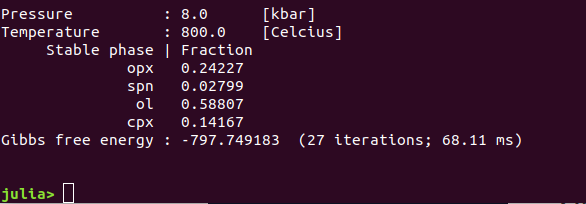
Access output structure
In Julia all the informations stored in the output structure stb_systems
can be listed by typing out.
and hitting the tab key twice tab + tab:
out.
which displays the content of structure out
:

The displayed informations are part of the C
output structure stb_systems
, and can be accessed individually (e.g., out.Gamma
) or displayed all at once using
print_info(out)
The full description of what contains the output structure is given in the CookBook: THERMOCALC-like output.
Examples of serial point calculation
#load MAGEMin
using MAGEMin_C
data = Initialize_MAGEMin("ig", verbose=false);
# One bulk rock for all points
P,T = 10.0, 1100.0
Xoxides = ["SiO2"; "Al2O3"; "CaO"; "MgO"; "FeO"; "Fe2O3"; "K2O"; "Na2O"; "TiO2"; "Cr2O3"; "H2O"];
X = [48.43; 15.19; 11.57; 10.13; 6.65; 1.64; 0.59; 1.87; 0.68; 0.0; 3.0];
sys_in = "wt"
out = single_point_minimization(P, T, data, X=X, Xoxides=Xoxides, sys_in=sys_in)
Finalize_MAGEMin(data)
for the metapelite database:
#load MAGEMin
using MAGEMin_C
#initialize
data = Initialize_MAGEMin("mp", verbose=false);
# provide bulk-rock composition
P,T = 2.0, 650.0
Xoxides = ["SiO2"; "Al2O3"; "CaO"; "MgO"; "FeO"; "Fe2O3"; "K2O"; "Na2O"; "TiO2"; "MnO"; "H2O"]
X = [69.64; 13.76; 1.77; 1.73; 4.32; 0.4; 2.61; 2.41; 0.80; 0.07; 10.0]
sys_in = "wt"
out = single_point_minimization(P, T, data, X=X, Xoxides=Xoxides, sys_in=sys_in)
Finalize_MAGEMin(data)
for the ultramafic database:
#load MAGEMin
using MAGEMin_C
#initialize
data = Initialize_MAGEMin("um", verbose=false);
# provide bulk-rock composition
P,T = 2.0, 650.0
out = single_point_minimization(P, T, data, test=0)
Finalize_MAGEMin(data)
Parallel point calculation Tutorial
To compute a list of single point calculation in parallel your can use the native Julia multi-threading. To activate multi-threading simply launch the Julia terminal as:
julia -t 4
or
julia --threads 4
where the number of threads depends on your system, generally twice the number of cores.
Examples of serial point calculation
To run n
points, using database ig
and test 0
(see List of pre-defined bulk-rock composition):
#load MAGEMin
using MAGEMin_C
#initialize
data = Initialize_MAGEMin("ig", verbose=false);
n = 100;
P = fill(8.0,n)
T = fill(800.0,n)
out = multi_point_minimization(P, T, data, test=1);
Finalize_MAGEMin(data)
Here the results are stored in out
as out[1:end]
. Various bulk-rock compositions can be prescribed as:
#load MAGEMin
using MAGEMin_C
#initialize
data = Initialize_MAGEMin("ig", verbose=false);
#set P-T-X conditions
P = [10.0, 10.0];
T = [1100.0, 1100.0];
Xoxides = ["SiO2"; "Al2O3"; "CaO"; "MgO"; "FeO"; "Fe2O3"; "K2O"; "Na2O"; "TiO2"; "Cr2O3"; "H2O"];
X1 = [48.43; 15.19; 11.57; 10.13; 6.65; 1.64; 0.59; 1.87; 0.68; 0.0; 3.0];
X2 = [49.43; 14.19; 11.57; 10.13; 6.65; 1.64; 0.59; 1.87; 0.68; 0.0; 3.0];
X = [X1,X2];
sys_in = "wt"
out = multi_point_minimization(P, T, data, X=X, Xoxides=Xoxides, sys_in=sys_in)
Other examples
Several additional tests are provided in ./test/runtests.jl
.